Freight Shipping¶
RocketShipIt is capable of creating freight shipments with Bill of Lading and other related documents. Here are a few examples:
UPS¶
Example:
<?php
$shipment = new \RocketShipIt\Freight('ups');
$shipment->setParameter('service', '308');
$shipment->setParameter('toCompany', 'John Doe');
$shipment->setParameter('toPhone', '1231231234');
$shipment->setParameter('toAddr1', '101 W Main');
$shipment->setParameter('toCity', 'Bozeman');
$shipment->setParameter('toState', 'MT');
$shipment->setParameter('toCode', '59715');
$shipment->setParameter('handleQty', '1');
$shipment->setParameter('handlePackageType', 'PLT');
// Repeat for multiple commodities
$c = new \RocketShipIt\Commodity('ups');
$c->setParameter('numberOfPieces', '10');
$c->setParameter('description', 'My fancy widgets!');
$c->setParameter('weight', '511.25');
$c->setParameter('length', '1.25');
$c->setParameter('width', '1.2');
$c->setParameter('height', '5');
$c->setParameter('commodityValue', '500');
$c->setParameter('packagingType', 'PKG');
$c->setParameter('freightClass', '55');
$c->setParameter('nmfcCode', '566');
$shipment->addCommodityLineToShipment($c);
// Optional services
$shipment->setParameter('callBeforeDelivery', '1');
$shipment->setParameter('constructionSite', '1');
$shipment->setParameter('holidayDelivery', '1');
$shipment->setParameter('insideDelivery', '1');
$shipment->setParameter('weekendDelivery', '1');
$shipment->setParameter('liftGateRequired', '1');
$shipment->setParameter('deliveryToDoor', '1');
$shipment->setParameter('limitedAccess', '1');
$shipment->setParameter('sortQty', '1');
$resp = $shipment->submitShipment();
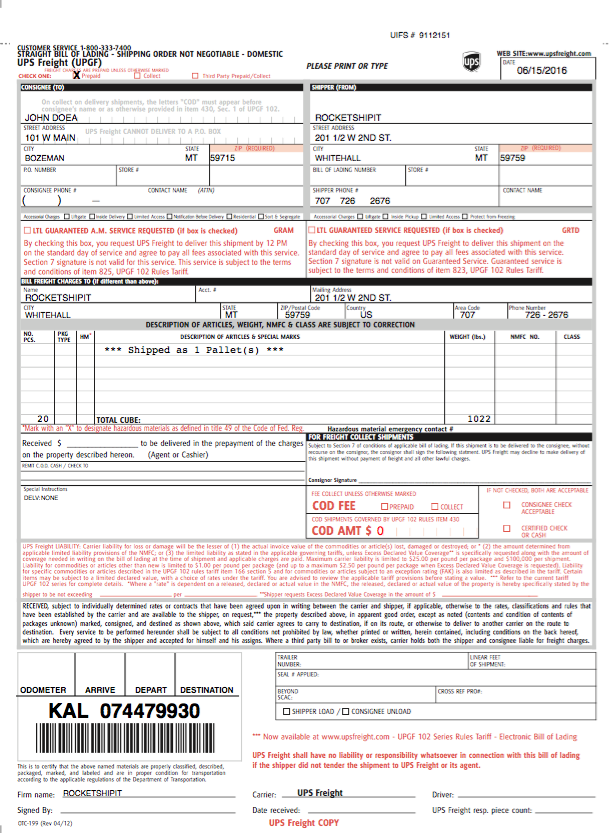
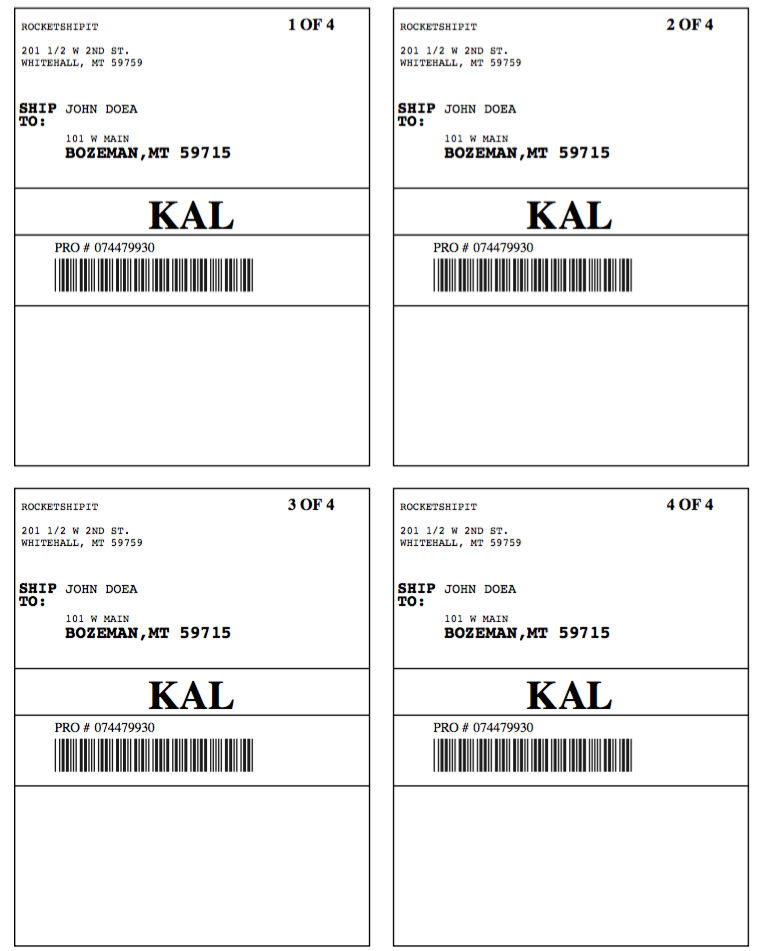
packagingType¶
BAG | Bag |
BAL | Bale |
BAR | Barrel |
BDL | Bundle |
BIN | Bin |
BOX | Box |
BSK | Basket |
BUN | Bunch |
CAB | Cabinet |
CAN | Can |
CAR | Carrier |
CAS | Case |
CBY | Carboy |
CON | Container |
CRT | Crate |
CSK | Cask |
CTN | Carton |
CYL | Cylinder |
DRM | Drum |
LOO | Loose |
OTH | Other |
PAL | Pail |
PCS | Pieces |
PKG | Package |
PLN | Pipe Line |
PLT | Pallet RCK Rack |
REL | Reel |
ROL | Roll SKD Skid |
SPL | Spool |
TBE | Tube TNK Tank |
UNT | Unit |
VPK | Van Pack |
WRP | Wrapped |
service¶
308 | UPS Freight LTL |
309 | UPS Freight LTL - Guaranteed |
334 | UPS Freight® LTL - Guaranteed A.M. |
349 | Standard LTL |
Sample Response¶
Example:
Array
(
[Meta] => Array
(
[Code] => 200
[ErrorMessage] =>
)
[Data] => Array
(
[Errors] => Array
(
[0] => Array
(
[Code] => 9369056
[Description] => No rates available.
[Type] => Warning
)
)
[Charges] => 0.00
[TrackingNumber] => 074480254
[ShipmentId] => 074480254
[Packages] => Array
(
)
[Documents] => Array
(
[0] => Array
(
[Code] => bill_of_lading
[Type] => application/pdf
[Media] => JVBERi0xLjUKJf...
)
[1] => Array
(
[Code] => label
[Type] => application/pdf
[Media] => JVBERi0xLjMKJf...
)
)
)
)